Difference between Abstract Class VS Interfaces
Abstract Class
- with keyword Abstract in the class Contain both abstract and non abstract methods. Abstract methods does not contain implementation in base class only contains method name with parameters with keyword abstract in the methods. Non Abstract methods contain implementation in the base Class itself.
- Abstract method should be override in the derived class by defining it.
- A class can be inherited from only one abstract class.
- Abstract class can't able to instantiate. It can be accessed through derived class objects.
- Abstract class have access specifiers for Function/Properties/Methods
Interface
- Interface is not a class it is a Entity defined by interfaces. Methods defined inside interface does not contain implementation only method name with parameter. Classes which inherited interfaces all methods defined will get Implemented in the base class.
- A class can have more than One Interfaces . It supports multiple inheritance.(C# does not support multiple inheritance.)
- Interface does not contain Access Specifier for Function/Properties/Methods default as Public.
INTERFACES OOPS Concept
All the classes will Inherit interfaces methods. Abstract classes serve some similar operation of interfaces. Inside the interface methods does not implemented. Interface will be define methods, properties and events
Interface is not a class it will represent with the key word 'interface'
Interface name contain with I prefix in the interface name.
Interface contain public as its modifiers.
Interface methods will be implemented in the class file which inherited interfaces.
A class can be Inherit more interfaces. Interfaces will support multiple inheritance.
code:
//Interface contain methods without implementation inside the interface
public interface Ivalue
{
string Name();
}
//Interface method declare in the inherited classes of the intefaces
Public class Operation : Ivalue
{
public string Name()
{
return "MANOJ KUMAR";
}
}
SAMPLE PROJECT
//Interface
public interface Isample
{
string fname
{
get;
set;
}
string lname
{
get;
set;
}
string Name();
}
//Class inherited with interfaces
public class Operation : Isample
{
protected string _fname;
protected string _lname;
public string fname
{
get
{
return _fname;
}
set
{
_fname = value;
}
}
public string lname
{
get
{
return _lname;
}
set
{
_lname = value;
}
}
public string Name()
{
return "First Name is " + fname + " & Last Name is " + lname;
}
}
class Program
{
static void Main(string[] args)
{
Isample sam = new Operation();
sam.fname = "Manoj";
sam.lname = "Kumar";
Console.WriteLine(sam.Name());
Console.ReadLine();
}
}
}
Result Set
Abstract Class
- with keyword Abstract in the class Contain both abstract and non abstract methods. Abstract methods does not contain implementation in base class only contains method name with parameters with keyword abstract in the methods. Non Abstract methods contain implementation in the base Class itself.
- Abstract method should be override in the derived class by defining it.
- A class can be inherited from only one abstract class.
- Abstract class can't able to instantiate. It can be accessed through derived class objects.
- Abstract class have access specifiers for Function/Properties/Methods
Interface
- Interface is not a class it is a Entity defined by interfaces. Methods defined inside interface does not contain implementation only method name with parameter. Classes which inherited interfaces all methods defined will get Implemented in the base class.
- A class can have more than One Interfaces . It supports multiple inheritance.(C# does not support multiple inheritance.)
- Interface does not contain Access Specifier for Function/Properties/Methods default as Public.
Abstract OOPS Concept
Abstract class contain both Abstract method and Non Abstract Method.Abstract class can not be instantiated. Abstract class identified using keyword "abstract" in the class prefix. Abstract class can be defined only with non abstract methods.
code:abstract class
abstract class Operation
{
//Abstract Method
public abstract int add(int num1,int num2);
//Non Abstract Method
public int sub(int num1, int num2)
{
return num1-num2;
}
public abstract int add(int num1,int num2);
//Non Abstract Method
public int sub(int num1, int num2)
{
return num1-num2;
}
}
Abstract method inside abstract class does not have implementation.
Abstract method can be identified using Keyword "abstract" in the method inside the abstract class.
Derived class of the abstract class contain the implementation of abstract methods.
Non Abstract method have implementation inside the abstract class.
Sample Abstract class Code
using System;
using System.Collections.Generic;using System.Text;
namespace AbstractClass
{
abstract class Operation
{
//Abstract Method
public abstract int add(int num1,int num2);
//Non Abstract Method
public int sub(int num1, int num2)
{
return num1-num2;
}
};
class Program : Operation
{
//Abstract method Override Operation in Derived Class
public override int add(int num1, int num2)
{
return num1 + num2;
}
static void Main(string[] args)
{
//Object of Derived class Instance created to access Methods
Program prog = new Program();
Console.WriteLine("addition:{0} Subtraction:{1}",prog.add(10, 5),prog.sub(50, 25));
Console.ReadLine();
}
}
}
Result Screen
Properties of Abstract class & methods:
- Abstract class can not be instantiated.
- Abstract method can not be declared as 'Private'
- Abstract class cant use sealed keyword.
- Abstract method cant use Virtual keyword.
- If the abstract method declares as Protected inside the abstract class In the derived class also abstract method with protected modifier.
- LEFT OUTER JOIN
- RIGHT OUTER JOIN
- FULL OUTER JOIN
- CROSS JOIN
- INNER JOIN
/*Create two tables*/
CREATE TABLE RightTable( ID [Int], Name Varchar(50));
GO
CREATE TABLE LeftTable(ID [INT], Amount[int]);
GO
/*Insert datas to the tables*/
INSERT INTO RightTable Values
(1,'man'),(2, 'sam'),(3,'jon'),(5,'joe'),(7,'kan')
GO
INSERT INTO LeftTable Values
(1,250),(2,550),(4,390),(6,874)
GO
/*LEFT JOIN*/
It returns all the rows in the Left side table and unmatched rows in the right side table will be "NULL"
SELECT *
FROM LeftTable
LEFT JOIN RightTable
ON LeftTable.ID=RightTable.ID
Result Set
/*RIGHT JOIN*/
It returns all the rows in the Right side table and unmatched rows in the Left side table will be "NULL"
SELECT *
FROM LeftTable
RIGHT JOIN RightTable
ON LeftTable.ID=RightTable.ID
Result Set
/*INNER JOIN*/
It returns only common rows in the both the tables.
SELECT *
FROM LeftTable
INNER JOIN RightTable
ON LeftTable.ID = RightTable.ID
Result Set
/*FULL JOIN*/
It returns all the rows from both the tables.
SELECT *
FROM LeftTable
FULL OUTER JOIN RightTable
ON LeftTable.ID = RightTable.ID
Result Set
/*CROSS JOIN*/
SELECT *
FROM LeftTable
CROSS JOIN RightTable
NORMALIZATION TECHNIQUE AND its RULES
What is normalization?
Database Design Technique used for avoiding redundant data and dependency data.
Before going to view the rules lets understand some basics,
Primary Key – unique identify for the database records without duplication.
Following Characteristics of Primary Key,
· Primary key does not allow null value
· Primary key must be unique
· Primary values cannot be changed
· Primary key created when new records inserted.
Composite Key – Combination of multicolumn values for identity a record unique.
Foreign Key –
Foreign Key - insert record in foreign key table will throw error if foreign Key doesn’t have entry in Primary Key.
Transitive Functional Dependencies
A transitive functional dependency is when changing a non-key column , might cause any of the other non-key columns to change
1NF - (no duplication of data/ single value for each column)
1NF Rules:
· Each column contain single value
· Each rows inserted must be unique
2NF – (Use of Referential integrity, Primary Key and Foreign Key)
2NF Rules
· Follow 1NF
· Single column Primary Key
3NF Rules
· Follow 2NF
· Has no transitive Functional Dependencies
Boyce-Codd Normal Form (BCNF)
Even when a database is in 3rd Normal Form, still there would be anomalies resulted if it has more than one Candidate Key.
Sometimes is BCNF is also referred as 3.5 Normal Form.
4th Normal Form
If no database table instance contains two or more, independent and multivalued data describing the relevant entity , then it is in 4th Normal Form.
5th Normal Form
A table is in 5th Normal Form only if it is in 4NF and it cannot be decomposed in to any number of smaller tables without loss of data.
The default process flow of request in asp.net web page
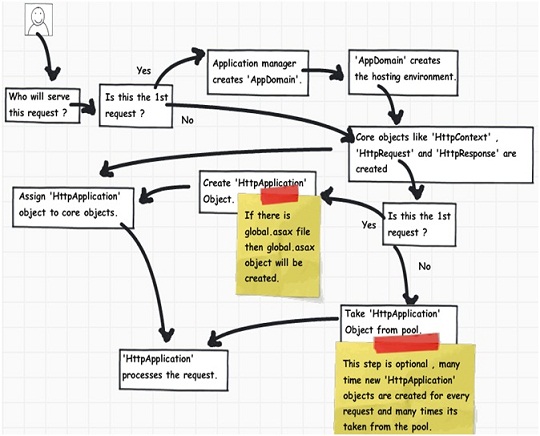
Client Request -> sent it to IIS and then request pass it to http module and then request sent to http handler -> page and again to HTTP Module.
M- HTTP Module - Which holds
Begin Request
Authenticate Request
Authorize Request
Resolve Request Cache
AcquireRequest state
PreRequest Handler
H-Handler- HTTP Handler-Process the request.
P-Page -(SILVERU)
S-Start
I-Init
L-Load
V-Validate
E- Events
R-Render
U-Unload
M -HTTP Module
PostRequestHandler
ReleaseRequestState
UpdateRequestCache
EndRequest
And Complete all the events fire Response will be displayed to Client.

The above thinks are need to remember during Asp.net Page Life Cycle.
Basic thinks to remember in Overloading, Overriding and Shadowing.
Overloading, Overriding and Shadowing
Overloading - Under single scope same method name used with multiple signature.
//Overloading
public class test
{
public void getStuff(int id)
{}
public void getStuff(string name)
{}
}
public class test
{
public void getStuff(int id)
{}
public void getStuff(string name)
{}
}
Overriding - Done by inheritance of the class modification in the base class.
Keyword - Override in derived class and virtual in base class.
//Overriding
public class test
{
public virtual getStuff(int id)
{
//Get stuff default location
}
}
public class test2 : test
{
public override getStuff(int id)
{
//base.getStuff(id);
//or - Get stuff new location
}
}
public class test
{
public virtual getStuff(int id)
{
//Get stuff default location
}
}
public class test2 : test
{
public override getStuff(int id)
{
//base.getStuff(id);
//or - Get stuff new location
}
}
Shadowing - Done by the inheritance of the class and maintain base class value.
Keyword - new in derived class.
//Shadowing
public class test
{
public getStuff(int id)
{
//Get stuff default location
}
}
public class test2 : test
{
public new getStuff(int id)
{
//base.getStuff(id);
//or - Get stuff new location
}
}
public class test
{
public getStuff(int id)
{
//Get stuff default location
}
}
public class test2 : test
{
public new getStuff(int id)
{
//base.getStuff(id);
//or - Get stuff new location
}
}
No comments :
Post a Comment